Spring Security - Getting Started
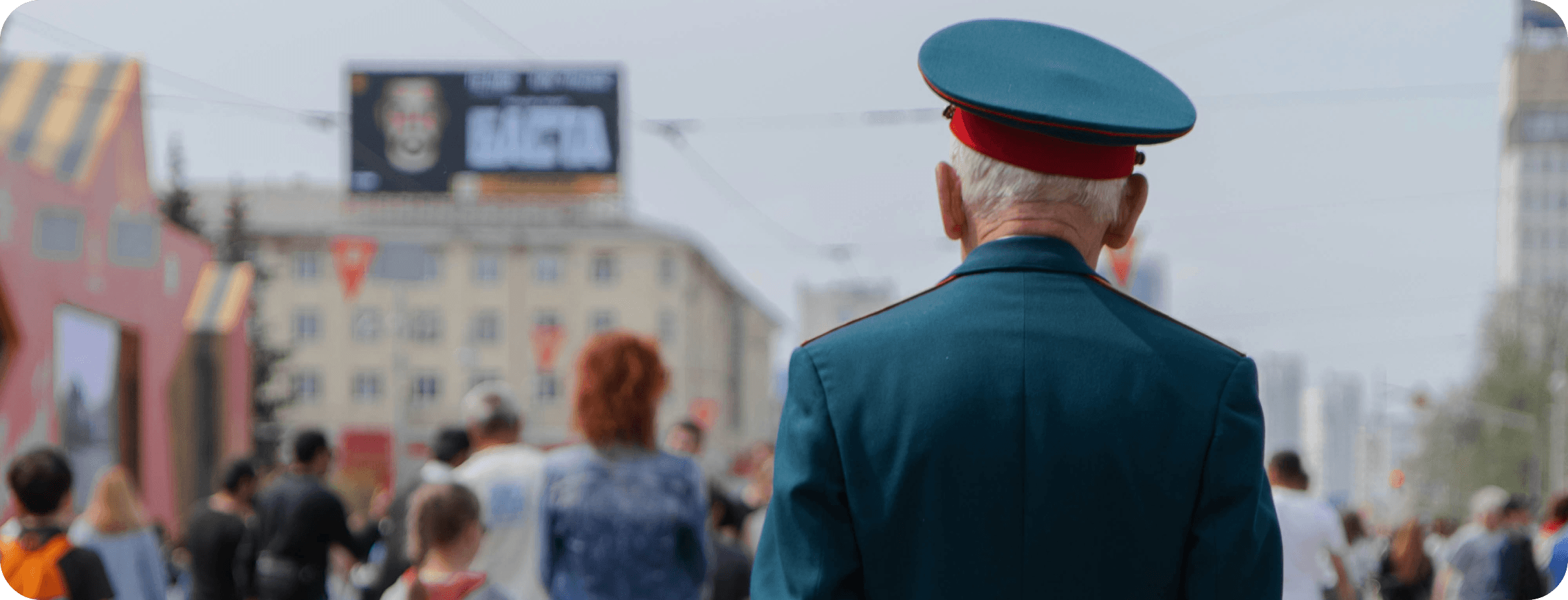
Features
Spring Security offers a robust set of features designed to secure web applications by addressing authentication and authorization needs. One of the key aspects of Spring Security is its ability to support various authentication methods, providing flexibility in how users are authenticated and ensuring the security of sensitive data 1.
Authentication
Authentication in Spring Security involves verifying the identity of users attempting to access protected resources or perform privileged actions. This is crucial for protecting sensitive information, ensuring privacy, preventing fraud, and building user trust 2. Spring Security facilitates different authentication mechanisms, each with distinct advantages and best practices 3.
Basic Authentication
Basic authentication is a straightforward method where user credentials (username and password) are sent in plain text with each request. While it is easy to implement and compatible with most web browsers, it is not very secure because the credentials can be intercepted by third parties. Therefore, it is recommended to use HTTPS to encrypt the credentials and mitigate security risks 4.
Form-Based Authentication
Form-based authentication requires users to provide credentials through a login form. This method is user-friendly, customizable, and allows centralized control over authentication processes. However, it is vulnerable to various attacks, such as phishing and cross-site scripting (XSS), making it essential to implement best practices like using HTTPS and enforcing secure password policies 4.
Token-Based Authentication
Token-based authentication involves generating a token, such as a JSON web token (JWT), upon successful authentication. The client uses this token for subsequent requests to access protected resources. This approach is stateless and scalable, providing a secure way to manage user sessions without relying on server-side sessions 3.
OAuth2
OAuth2 is an open standard that enables users to grant third-party applications access to their resources without sharing their credentials. It is widely adopted due to its ability to support modern authentication scenarios, such as social login and API integration, making it a versatile choice for securing web applications 3.
Getting Started
This guide introduces you to the process of creating a simple web application secured by Spring Security 5. You will build a Spring MVC application that includes a login form, backed by a fixed list of users, to protect certain resources 5. The project begins with creating an unsecured web application, followed by adding security measures to protect it.
To initiate the project, you can either start from scratch or skip the basic setup if you are already familiar with it 5. Starting from scratch involves using Spring Initializr, while skipping the basics allows you to directly compare your results with the complete code provided in gs-securing-web/complete 5.
Initially, you will develop a web application with two simple views: a home page and a "Hello, World" page. These pages are defined using Thymeleaf templates in home.html
and hello.html
, respectively 5. The application leverages Spring MVC, requiring you to configure Spring MVC and set up view controllers to expose these templates. This is achieved by using the MvcConfig
class, which adds view controllers for the home and hello pages, as well as a login page that will be created in the subsequent steps 5.
Once the basic web application is set up, Spring Security is introduced to prevent unauthorized access to the greeting page at /hello
5. By adding Spring Security to the classpath and configuring it properly, all HTTP endpoints are secured with basic authentication by default 5. The configuration is further customized to allow unrestricted access to the home page while requiring authentication for all other paths 5.
The security configuration is managed through the WebSecurityConfig
class, which defines URL security and sets up an in-memory user store 5. This configuration ensures that only authenticated users can access restricted pages, redirecting them to a custom login page if they attempt to access secure content without logging in 5.
With the application configured and the security measures in place, you can run the application either using Gradle or Maven, and deploy it as an executable JAR file for easy distribution and deployment across different environments 5. Once the application is running, accessing http://localhost:8080
in a browser will display the home page, and attempting to navigate to the greeting page will prompt a login, demonstrating the implemented security features 5.
Configuration
Configuring Spring Security involves understanding its core architecture and properly setting up authentication and authorization mechanisms to secure applications built with Java Spring Boot. A fundamental aspect of this configuration is the use of filter chains, which manage the processing of incoming requests before they reach the handler 6.
Spring Security's configuration can be broken down into several packages, each handling different security aspects. For instance, the org.springframework.security.config.annotation.web.configuration
package is pivotal for configuring web-based security, while org.springframework.security.config.annotation.authentication.configurers.userdetails
focuses on setting up user details for authentication 7. These configurations are typically done in a Java configuration file where developers can specify security constraints, authentication mechanisms, and other relevant security settings 8.
For a typical Spring Boot application, developers may start by implementing a custom AuthenticationManager
to delegate authentication responsibilities, especially when integrating external services or custom authentication logic 8. This can involve configuring Spring Security to use this manager to authenticate users based on a REST API response from an existing application 8.
Moreover, configuring JWT tokens for authorization and potentially integrating OAuth as a secondary login option can enhance security. The packages like org.springframework.security.oauth2.client
and org.springframework.security.oauth2.server.resource
provide essential support for OAuth 2.0 client and resource server functionalities 9.
In addition to these core configurations, developers might need to create custom filters to handle specific security requirements, such as Attribute-Based Access Control (ABAC) 10. This is achieved by defining custom authorization filters within the Spring Security context, allowing for fine-grained access control over application endpoints 10.
Core Components
Spring Security is an extensive framework designed to manage access control in Java applications, and it is comprised of numerous core components that enable its powerful security features. These components are organized into various packages, each catering to specific aspects of security requirements.
One of the primary packages is org.springframework.security.access
, which handles core access-control related code including security metadata, interception code, and access control annotations. It also supports expression language (EL) and voter-based implementations for the central AccessDecisionManager
interface 7. This package ensures that proper authorization decisions are made based on configured security constraints.
For handling authorization events, org.springframework.security.access.event
provides necessary classes and interfaces. These events can be used to monitor and respond to authorization activities within the application 7. Additionally, the org.springframework.security.access.intercept
package includes abstract level security interception classes, crucial for enforcing the configured security constraints on secure objects 7.
Another significant aspect of Spring Security is its support for user authentication and authorization. The org.springframework.security.core
package contains core classes and interfaces related to user authentication and authorization, as well as the maintenance of a security context 7. This package forms the backbone of user identity management and role-based access control within the framework.
In terms of web security, org.springframework.security.web
is the central module providing necessary tools to secure web applications. It encompasses a variety of packages dedicated to different security needs, such as org.springframework.security.web.authentication
for processing authentication credentials using various protocols, and org.springframework.security.web.access
for managing access-control related classes and packages 7.
Furthermore, Spring Security extends its capabilities to support modern authentication mechanisms like OAuth 2.0 and OpenID Connect. This is facilitated through packages such as org.springframework.security.oauth2.client
and org.springframework.security.oauth2.core
, which provide the necessary classes and interfaces for implementing OAuth 2.0 client and authorization server interactions 7.
These core components and their corresponding packages offer a robust foundation for developers to build secure applications, ensuring that both authentication and authorization are effectively managed within the Spring ecosystem.
Customization
Spring Security provides robust mechanisms for customizing authentication and authorization, enabling developers to tailor security to specific application requirements. A common customization involves creating a custom authentication provider by implementing the AuthenticationProvider
interface. This allows for integrating bespoke authentication logic directly into the Spring Security framework 11.
Custom Authentication Filter
For scenarios that require a distinct authentication process, such as validating custom HTTP headers, a custom authentication filter can be implemented. Developers may opt to create a filter that processes incoming requests to construct a UsernamePasswordAuthenticationToken
based on custom headers, like "foo_username" and "foo_password" 12 13. This approach entails managing authentication errors, such as missing headers or incorrect passwords, and determining when to delegate down the filter chain without altering the security context 12.
When creating a custom filter, it is crucial to decide between implementing interfaces like Filter
, OncePerRequestFilter
, or AbstractAuthenticationFilter
. Each option provides different levels of control over the filtering process and integration with Spring's security context 13. The authentication responsibilities can be divided among various components, with the AuthenticationManager
playing a pivotal role in managing authentication processes 12.
Packages and Interfaces
Spring Security offers a wide range of packages to support customization efforts. For instance, the org.springframework.security.access
package encompasses core access-control-related code, while the org.springframework.security.web.authentication
package provides mechanisms for authentication processing 7. These packages collectively offer a foundation for developing customized security solutions.
Authorization and Access Control
Customization isn't limited to authentication. Developers may also implement custom authorization filters or adapt existing ones to support Attribute-Based Access Control (ABAC). This involves leveraging security metadata and voter-based implementations to tailor access decisions based on dynamic attributes 10. The org.springframework.security.access
package, with its security annotations and expression language (EL) support, provides the necessary tools for such authorization customizations 7.
Testing
Testing is an essential component of developing secure applications with Spring Security. It involves validating that the implemented security measures function correctly and that the application is protected against various vulnerabilities.
Vulnerability Testing
To ensure that a Spring Security application is resilient against known vulnerabilities, developers can use tools such as Tenable Web App Scanning. This dynamic application security testing (DAST) tool scans running web applications to detect vulnerabilities such as cross-site scripting (XSS) and SQL injection, which are part of the OWASP Top 10 vulnerabilities 14. By using Tenable Web App Scanning, developers can identify vulnerabilities in both custom application code and third-party components, allowing for comprehensive vulnerability coverage.
Secure Coding Practices Testing
Testing the secure coding practices implemented in a Spring Security application involves verifying that key security controls are correctly configured and functioning as intended. For instance, input validation should be tested to ensure that user-provided inputs are properly sanitized and validated using techniques such as server-side validation and input sanitization libraries 15. Furthermore, the security of SQL queries should be validated to confirm that they are protected against SQL injection attacks by using parameterized queries or similar methods 15.
Authentication and Authorization Testing
It is critical to test authentication and authorization mechanisms to ensure that they correctly restrict access to resources based on user roles and permissions. Spring Security offers annotations such as @PreAuthorize
to control method access based on roles, which should be tested to verify that only authorized users can access certain features 15. Additionally, testing should ensure that session management is secure to prevent session hijacking and other related attacks.
Continuous Testing
Continuous testing is a best practice for maintaining application security over time. Tools like Veracode can be used for continuous vulnerability scanning, ensuring that any new vulnerabilities introduced by updates or changes are promptly identified and mitigated 15. This ongoing testing process helps maintain the integrity, confidentiality, and availability of the application and its data.
Security Best Practices
When implementing application security, following best practices is crucial to ensuring robust protection against potential threats. Spring Security offers several features that facilitate adherence to these best practices with minimal configuration.
Emphasize Secure Authentication
Authentication is a critical component of application security. It involves verifying the identity of a user, typically using knowledge-based authentication like usernames and passwords. However, relying solely on this method can be risky if credentials are compromised. It is advisable to implement multi-factor authentication, which combines knowledge-based and possession-based authentication methods, such as one-time passwords (OTPs) sent to a user's mobile device. This layered approach significantly enhances security by ensuring that only users who possess specific items or information can gain access 16.
Implement Granular Authorization
Authorization ensures that authenticated users have the correct permissions to perform specific actions within an application. It is essential to define clear access control policies and implement them using granted authorities and roles. Spring Security provides a robust mechanism to manage these permissions, allowing developers to assign granular authorities to users or group them into roles. For instance, different roles like ADMIN or USER can be configured to grant specific access rights, streamlining permission management and reducing the risk of unauthorized access 16.
Regularly Update Dependencies
Security vulnerabilities are frequently discovered in software dependencies, which can leave applications exposed to attacks. It is crucial to keep all dependencies up-to-date, ensuring that any known vulnerabilities are patched. Spring Security helps mitigate these risks by handling many common vulnerabilities automatically. By integrating Spring Security, developers can focus more on application features while trusting that the framework is providing a solid security foundation 16.
Use Filters Wisely
Filters play a vital role in processing requests before they reach the application. They can be used to perform various tasks, such as input validation, logging, and encryption, which enhance security. Spring Security provides a series of filters that examine each request, allowing or denying access based on pre-defined rules. Properly configuring these filters is essential for maintaining a secure application environment 16.
Secure Default Configurations
Out-of-the-box, Spring Security offers a set of default configurations that enforce mandatory authentication for all URLs and provide a basic login form. While these defaults provide a secure starting point, developers should customize them to meet specific application requirements. This customization might include specifying authentication methods, tailoring login pages, or defining error-handling strategies to suit the application's context. These adjustments ensure that security measures align with the application's specific needs 16.
Troubleshooting
When working with Spring Security, developers may encounter various issues that require troubleshooting. One common problem arises when custom authentication providers are introduced into the security configuration. If a custom authentication provider is declared using the @Component
annotation, it might not be automatically detected by Spring Security's BasicAuthenticationFilter
, leading to authentication failures 17.
A typical error encountered in such scenarios is the ProviderNotFoundException
, which occurs when no AuthenticationProvider
is found for a specific authentication token, such as UsernamePasswordAuthenticationToken
17. This exception indicates that Spring Security was unable to locate a suitable provider to authenticate the request.
To resolve this, ensure that the custom authentication provider is correctly configured and integrated into the Spring Security filter chain. Custom filters, like CustomFilter
, can be used to handle specific authentication logic before delegating to the standard authentication mechanisms 17. This involves overriding the doFilterInternal
method to implement the desired authentication behavior and update the security context accordingly.
Another potential issue involves handling complex authorization scenarios where role-based or authority-based checks are insufficient. In such cases, customizing access control rules to account for specific conditions not covered by default mechanisms can be essential 18. This may involve implementing custom AccessDecisionVoter
s or using expressions in security configuration to evaluate complex conditions.
When troubleshooting these issues, detailed logging can be invaluable. By increasing the logging level for Spring Security components, developers can gain insights into the filter chain processing and identify where the authentication or authorization process is failing 17. Understanding the sequence and configuration of filters, as well as how custom components integrate into the existing security framework, is crucial for effective problem-solving.
Community and Support
Spring Security benefits from an active and robust community that contributes to its ongoing development and provides extensive support resources for users. The community is engaged in discussions through various forums and online platforms, such as Stack Overflow and GitHub, where users can seek advice, share solutions, and collaborate on improvements and bug fixes 2 3. Additionally, the official Spring Security documentation is a comprehensive resource, offering detailed guides and reference materials to assist developers in understanding and implementing security measures in their applications 19 1.
Users can also find support through numerous blogs, webinars, and video tutorials produced by the community and Spring experts, providing practical insights and hands-on examples of using Spring Security effectively 20 21. Furthermore, the Spring Security team regularly updates the framework with new features and security patches, ensuring that users have access to the latest security standards and practices 7 22. For developers seeking more personalized support, commercial options are available through Spring's parent company, VMware, which offers professional support services and consulting 23 24.
Mitigating Security Vulnerabilities
Spring Security is an essential framework for mitigating security vulnerabilities in applications, offering a comprehensive suite of tools and configurations that simplify the implementation of robust security features 16. It addresses common security challenges by managing authentication and authorization processes, reducing the risk of unauthorized access and data breaches 16.
One of the core strengths of Spring Security is its ability to handle common vulnerabilities, which alleviates the burden on developers who might otherwise struggle with constantly evolving security threats 16. By integrating seamlessly with other frameworks like Spring MVC, Spring Security provides a flexible and customizable security solution that adapts to specific application needs, ensuring that developers can focus on enhancing functionality while maintaining high security standards 16.
Spring Security also incorporates a range of authentication mechanisms, including knowledge-based and possession-based authentication, which enhance user verification processes 16. Multi-factor authentication, combining both types, is supported to further strengthen security 16. Authorization in Spring Security is managed through roles and granted authorities, which define user permissions and help prevent unauthorized actions within the application 16.
Moreover, Spring Security's filter chain mechanism scrutinizes each request to ensure that only authenticated and authorized users gain access to application resources 16. This approach includes pre-configured filters that handle tasks such as credential validation and error management, streamlining the security process without requiring extensive manual configuration 16.
Advanced Concepts
Spring Security is a comprehensive security framework for Java applications, offering a wide range of features and options for securing applications. A critical part of its architecture is based on the use of standard Servlet filters, which are employed to process HTTP requests and responses 25. These filters are organized into a FilterChain, allowing multiple filters to be applied to a single request. The order of the filters is crucial because each filter may depend on the outcome of its predecessors 26.
One of the key components of Spring Security is the concept of GrantedAuthority
, which is used to store the authorities that have been granted to a user. These are typically inserted into the Authentication
object by an AuthenticationManager
and later read by AccessDecisionManager
instances when making authorization decisions 22. Spring Security includes a concrete implementation, SimpleGrantedAuthority
, which allows user-specified strings to be converted into authorities. This implementation is commonly used with role-based authorization, where the prefix "ROLE_" is often employed 22.
Another advanced feature of Spring Security is the DelegatingFilterProxy
, which acts as a proxy for a standard Servlet filter. This proxy delegates calls to a Spring-managed bean implementing the Filter
interface, enabling the application of the full Spring bean definition machinery to complex filter implementations 27. This setup is particularly beneficial for filters that require intricate configuration, allowing developers to leverage Spring's capabilities for managing bean lifecycles and dependencies 27.
The flexibility of Spring Security extends to its support for different authentication and authorization methods, such as JWT tokens and OAuth. These methods can be integrated into the security framework to provide more robust security measures for REST APIs and other application types 9. The security configuration can be customized further by adjusting the filter chain and incorporating additional filters or authentication mechanisms as needed 26. This allows developers to tailor the security setup to meet specific application requirements while maintaining a high level of protection against common security threats.
In conclusion, Spring Security provides a comprehensive framework for securing Java applications, offering robust features for authentication and authorization.

Start Your Cybersecurity Journey Today
Gain the Skills, Certifications, and Support You Need to Secure Your Future. Enroll Now and Step into a High-Demand Career !
Fusion Cyber Blogs
RECENT POSTSThe Future of AI & Cyber Reskilling is Here – Are You Ready?
Fusion Cyber is revolutionizing workforce training with AI-powered reskilling to boost efficiency and security.
Read MoreU.S. Coast Guard's New Cyber Rule: What Maritime Firms Must Know
The U.S. Coast Guard's new cybersecurity rule, effective July 16, 2025, mandates stricter cyber protections for maritime organizations, including risk assessments, incident response plans, and a designated Cybersecurity Officer.
Read More